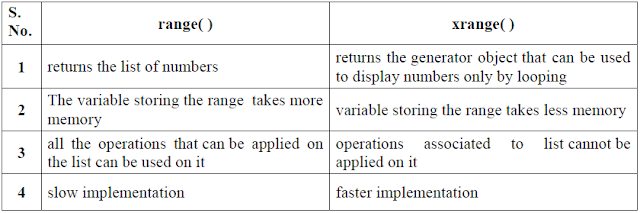
The range( ) function
- It generates a list of numbers, which is generally used to iterate over with for loop.
- range( ) function uses three types of parameters, which are:
- start: Starting number of the sequence.
- stop: Generate numbers up to, but not including last number.
- step: Difference between each number in the sequence.
Python use range( ) function in three ways:
- range(stop)
- range(start, stop)
- range(start, stop, step)
Note:
- All parameters must be integers.
- All parameters can be positive or negative.
1. range(stop): By default, It starts from 0 and increments by 1 and ends up to stop, but not including stop value.
Example:
for x in range(4):
print(x)
Output:
0
1
2
3
2. range(start, stop): It starts from the start value and up to stop, but not including stop value.
Example:
for x in range(2, 6):
print(x)
Output:
2
3
4
5
3. range(start, stop, step): Third parameter specifies to increment or decrement the value by adding or subtracting the value.
Example:
for x in range(3, 8, 2):
print(x)
Output:
3
5
7
Explanation of output:
3 is starting value, 8 is stop value and 2 is step value. First print 3 and increase it by 2, that is 5, again increase is by 2, that is 7. The output can’t exceed stop-1 value that is 8 here. So, the output is 3, 5, 8.